How to Build a Faceless Tiktok Channel with AI
Building a faceless video channel is an increasingly popular trend in content creation, especially on platforms like TikTok and YouTube. It allows creators to produce engaging content without ever showing their face on camera.

Sam Bowen-Hughes
Creator
Building a faceless video channel is an increasingly popular trend in content creation, especially on platforms like TikTok and YouTube. It allows creators to produce engaging content without ever showing their face on camera, leveraging the power of AI to streamline content production, editing, and even voiceover. In this post, we'll explore how AI tools can help you create high-quality, faceless video content and why this strategy is perfect for anyone looking to grow a video channel without stepping in front of the camera.
Why a Faceless Video Channel?
Faceless video channels provide a variety of benefits:
- Privacy: You can maintain anonymity, which is great for creators who are camera-shy or want to keep their personal life separate from their content.
- Efficiency: AI tools help you automate large parts of the video creation process, from editing to adding voiceovers, meaning you can focus more on creativity and scaling your content.
- Niche Focus: Without the need to show your face, you can focus entirely on delivering value through niche topics like education, tutorials, or entertainment without worrying about on-screen performance.
Step 1: Generating Ideas and Scripts with AI
The first step to building a faceless video channel is coming up with engaging ideas and scripts. AI models like ChatGPT can help brainstorm content ideas and even write scripts tailored to your audience.
For example, if you're building a niche channel about personal finance, you could ask GPT to generate script ideas based on trending topics:
"Generate 5 script ideas for a TikTok channel about saving money on groceries."
You’ll instantly get ideas and detailed outlines for quick TikToks that grab attention and provide value. The AI can also adapt scripts to fit various video lengths or formats, ensuring you’re consistently delivering content in a way that resonates with your target audience.
Step 2: Creating Visuals with AI-Generated Content
Faceless channels thrive on visual storytelling, and AI makes it easier than ever to create compelling visuals without showing yourself. Tools like DALL·E and Midjourney can generate custom images, illustrations, and even short animations to bring your content to life.
For TikTok, you can use AI-generated images to create explainer videos, tutorials, or story-based content. Let’s say you’re making a video on “5 best side hustles in 2024”; instead of showing your face, you can use illustrations or visual cues generated by AI to represent each side hustle. This not only keeps the content engaging but also taps into the growing trend of faceless creators on TikTok.
Step 3: Using AI Voiceovers for Seamless Narration
One of the essential aspects of faceless content is the audio, especially narration. AI-powered voiceover tools like Descript and WellSaid Labs enable you to create human-like voiceovers for your videos without needing to record your own voice.
You can type in your script, and these tools will generate a high-quality voiceover. The ability to choose different voice styles, accents, and tones allows for even more customization, making your content feel unique. This is a fantastic time-saver for creating faceless TikTok channels where rapid content production is key to growth.
Step 4: Automating Video Editing with AI
Once you have your visuals and voiceovers ready, the next step is putting it all together. AI-powered video editing tools like Pictory, Kapwing, or even custom setups with Remotion can streamline the entire editing process. These tools allow you to:
- Auto-sync voiceovers with visuals: Instead of manually syncing audio with clips, AI can automatically match them up for you.
- Automatically trim and cut clips: Tools like Pictory analyze video content and suggest or even apply cuts to make your content more engaging and concise.
- Add AI-generated effects and transitions: AI editors can automatically add transitions, effects, and music, saving you hours of manual work.
Using Remotion with React and Tailwind CSS, you can even build your own custom editing pipeline tailored specifically to your channel’s needs, making it easier to apply consistent editing styles across all videos.
Step 5: Optimizing for TikTok with AI
Creating faceless video content is just one part of the equation. You’ll also want to optimize your content for maximum reach. This is where AI analytics tools come into play. Platforms like TubeBuddy and VidIQ can help you identify trending hashtags, topics, and keywords that will get your videos seen by more people.
AI can even suggest the best times to post, ensuring your content gets the most engagement when your audience is active.
Benefits of a Faceless TikTok Channel
Now that you understand the technical steps, let’s talk about why this strategy is so effective:
-
Scalability: Once your process is set up, you can automate large parts of content production, allowing you to create and post consistently without spending hours editing each video.
-
Creative Freedom: Without being on camera, you can focus more on creativity and delivering unique content rather than worrying about appearance or performance.
-
Monetization Opportunities: Faceless channels are just as viable for monetization through sponsorships, affiliate marketing, and TikTok’s creator fund. Plus, many brands prefer content that focuses on their product or message without a personal face attached to it.
-
Rapid Content Production: With AI handling scripts, editing, and voiceovers, you can pump out high-quality content at a much faster rate. This is perfect for platforms like TikTok, where consistency is crucial to growing a large audience.
Conclusion: Start Your Faceless Video Channel with AI Today
AI tools have made it easier than ever to create faceless video content. Whether you’re starting a TikTok channel or a YouTube series, leveraging AI for scriptwriting, visuals, voiceovers, and editing can save you time, increase the quality of your content, and help you grow your audience quickly.
Want a powerful video editor to help you get started? Check out React Video Editor Pro. We’ve done the hard part for you, so you can focus on building the next big faceless TikTok channel.
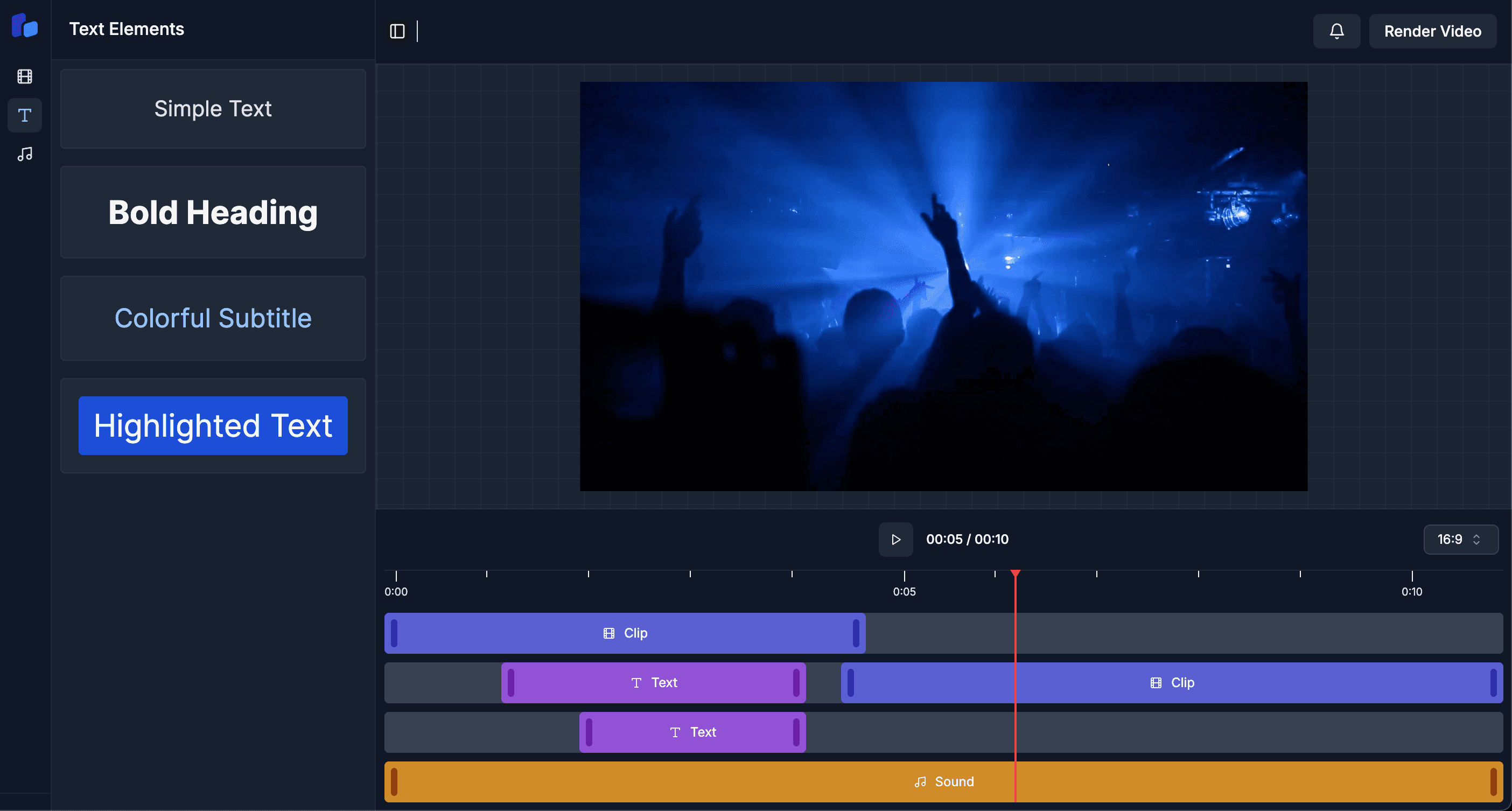
Create Your Own Video Editor in Minutes with RVE
Theres never been a better time to build.Keep Reading
Explore more related articles
Previous Article
Why I Created the React Video Editor
Discover why I built a React Video Editor component, leveraging Remotion and Next.js, to simplify the complex process of video editing in web apps.
Next Article
How to Build an AI-Powered Video Editor
AI-powered video editors are revolutionizing the world of content creation, enabling creators to automatically enhance and transform videos with minimal effort.