Understanding Overlays in React Video Editor
Overlays are the backbone of video composition in React Video Editor. Let's dive into how they work and how you can use them to create structured, reusable video templates.
Sam
Creator or RVE
Overlays are the core building blocks of React Video Editor, defining every element that appears in a video whether it’s text, images, video clips, shapes, sound, or captions. They determine when elements appear, how they look, and how they interact with other layers on the timeline.
Each overlay is an independent unit, but together they form a structured timeline where different elements layer, animate, and interact to create the final composition. Once you understand how overlays work, you have full control over assembling videos dynamically.
How Overlays Work
At their simplest, overlays are objects that hold information about a media element. This includes:
- Type – What kind of overlay it is (text, image, video, etc.).
- Position – Where it appears on the canvas.
- Duration – How long it stays visible.
- Styles – Fonts, colors, opacity, effects, and animations.
- Source Data – A URL or file reference for media elements.
For example, a text overlay that appears in the middle of a video might look like this:
{ id: 1, type: OverlayType.TEXT, content: "Hello, world!", from: 50, durationInFrames: 100, styles: { fontSize: "2rem", color: "#FFFFFF", textAlign: "center", animation: { enter: "fade", exit: "fade" } } }
This means:
- The text "Hello, world!" will appear at frame 50.
- It will remain on screen for 100 frames.
- It will be white, centered, and fade in and out.
The same concept applies to videos, images, and sounds, except each type has its own unique properties.
Different Types of Overlays
Text Overlays
Used for adding titles, subtitles, captions, or dynamic typography.
{ id: 2, type: OverlayType.TEXT, content: "New Update", from: 30, durationInFrames: 80, styles: { fontSize: "3rem", fontWeight: "bold", color: "#FFD700" } }
Image Overlays
Allows placement of images on the video, such as logos, watermarks, or background graphics.
{ id: 3, type: OverlayType.IMAGE, src: "https://example.com/image.png", from: 0, durationInFrames: 150, styles: { objectFit: "contain", width: 200, height: 100 } }
Video Overlays
Embeds clips inside the main composition, allowing for multi-layered video projects.
{ id: 4, type: OverlayType.VIDEO, src: "https://example.com/video.mp4", from: 0, durationInFrames: 300, styles: { objectFit: "cover" } }
Shape Overlays
Basic rectangles, circles, or other geometric elements that add visual structure.
{ id: 5, type: OverlayType.SHAPE, content: "rectangle", from: 10, durationInFrames: 120, styles: { fill: "#FF0000", borderRadius: "8px", opacity: 0.8 } }
Sound Overlays
Controls music, voiceovers, or sound effects.
{ id: 6, type: OverlayType.SOUND, src: "https://example.com/audio.mp3", from: 0, durationInFrames: 200, styles: { volume: 0.7 } }
Caption Overlays
Used for subtitles and on-screen dialogue.
{ id: 7, type: OverlayType.CAPTION, from: 146, durationInFrames: 200, captions: [ { text: "Welcome to the tutorial", startMs: 0, endMs: 3000 } ], styles: { fontSize: "1.5rem", color: "#FFFFFF", textAlign: "center" } }
Bringing It All Together
Since overlays exist as structured objects, they can be dynamically combined to build complex video compositions. A single scene might contain:
- A video background.
- An animated title text.
- A lower-third image overlay.
- A soundtrack playing underneath.
- A subtitle track for accessibility.
Each of these layers is defined separately, but together they create a fully structured video.
Example: A Complete Scene
const scene: Overlay[] = [ { id: 1, type: OverlayType.VIDEO, src: "https://example.com/main-video.mp4", from: 0, durationInFrames: 500, styles: { objectFit: "cover" }, }, { id: 2, type: OverlayType.TEXT, content: "Welcome to the Show", from: 30, durationInFrames: 100, styles: { fontSize: "3rem", fontWeight: "bold", color: "#FFFFFF", textAlign: "center", animation: { enter: "fade", exit: "fade" }, }, }, { id: 3, type: OverlayType.IMAGE, src: "https://example.com/logo.png", from: 0, durationInFrames: 500, styles: { objectFit: "contain", width: 100, height: 50, top: 20, left: 20 }, }, { id: 4, type: OverlayType.SOUND, src: "https://example.com/background-music.mp3", from: 0, durationInFrames: 500, styles: { volume: 0.5 }, }, ];
This defines a structured composition where elements are layered on top of each other.
Extending Overlays
Overlays are just data structures, meaning they can be extended with new properties.
If you need additional styles, animations, or interactivity, you can modify the type definitions.
For example, if you wanted to support border and shadow effects for text overlays, you could update the type model:
export type ExtendedTextOverlay = TextOverlay & { styles: TextOverlay["styles"] & { border?: string; boxShadow?: string; }; };
Now you can define text overlays with extra styling:
{ id: 8, type: OverlayType.TEXT, content: "Breaking News", from: 20, durationInFrames: 120, styles: { fontSize: "2.5rem", fontWeight: "bold", color: "#FFFFFF", border: "2px solid red", boxShadow: "4px 4px 10px rgba(0, 0, 0, 0.5)" } }
There are no limits to what overlays can do. If you need drop shadows, motion effects, perspective transformations, or new types of elements, it's just a matter of extending the model.
Templates as a Natural Extension
Once you understand overlays, the next step is grouping them into reusable templates.
Instead of creating each overlay manually, templates allow you to predefine entire video structures.
For example, a social media post template might always include:
- A background video.
- A logo in the top corner.
- A text overlay with a call to action.
- A background music track.
By defining these as a template, they can be applied dynamically to multiple projects.
The Takeaway
Overlays are the foundation of video composition. They define what appears on screen, how it looks, and when it happens. Whether it's a simple text label or a fully animated title sequence, everything in the React Video Editor is built on overlays.
The system is fully extendable, allowing you to add new styles, animations, or even entirely new types of overlays. Once you understand how overlays fit together, the possibilities for structuring and automating video creation are endless.
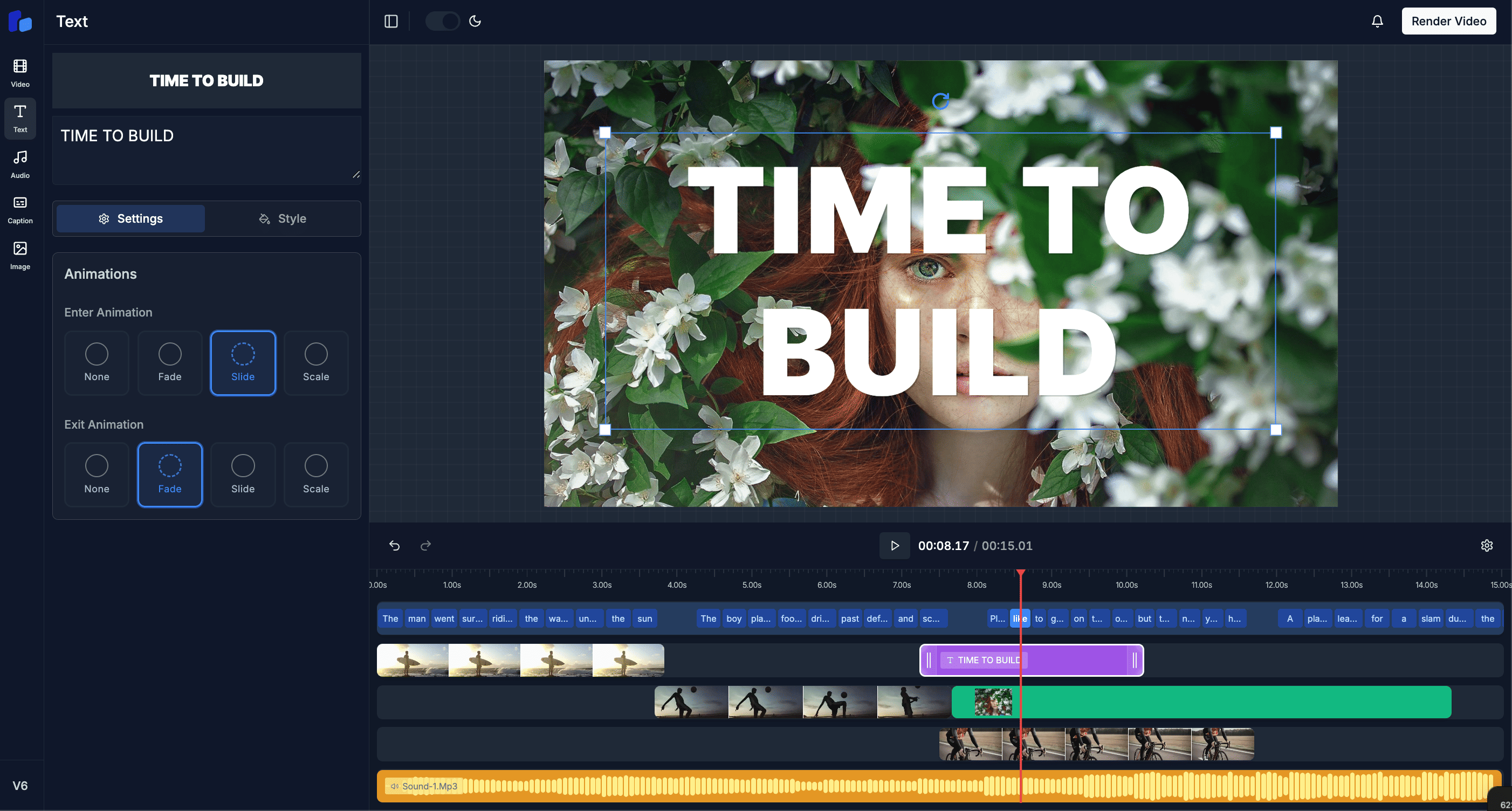
Ready to Build YourNext Video Project?
Join developers worldwide who are already creating amazing video experiences. Get started with our professional template today.
Keep Reading
Explore more related articles